Intro
Master Cpp calendar management with 5 expert tips, boosting productivity via efficient scheduling, date manipulation, and time organization techniques.
Creating a calendar in C++ can be a complex task, but with the right approach, it can be accomplished efficiently. Calendars are essential tools for organizing and managing time, and programming them requires a solid understanding of date and time manipulation. In this article, we will delve into the world of C++ calendar programming, exploring five valuable tips to help you create and utilize calendars effectively in your applications.
The importance of calendars in programming cannot be overstated. They are used in a wide range of applications, from simple reminders and schedulers to complex systems for managing appointments, events, and tasks. Understanding how to work with dates and times in C++ is crucial for developing applications that require temporal awareness. Whether you're a beginner or an experienced programmer, mastering calendar-related functionalities can significantly enhance your programming skills and the usability of your applications.
Before we dive into the tips, it's essential to understand the basics of how C++ handles dates and times. The C++ Standard Library provides the <ctime>
header, which includes functions for manipulating dates and times. However, for more complex operations, such as calculating the day of the week or determining leap years, you may need to implement your own functions or use third-party libraries. The key to successfully working with calendars in C++ is to have a solid grasp of these fundamental concepts and to approach calendar-related tasks with a clear, structured methodology.
Understanding the C++ Date and Time Library

To effectively work with calendars in C++, you need to understand the <ctime>
library and its components. This library provides a set of functions for converting between different time representations, such as converting a time_t
object (which represents the number of seconds since the epoch, January 1, 1970) to a struct tm
(which breaks down time into year, month, day, hour, minute, and second). Mastering the conversion between these representations is vital for performing calendar operations.
Key Functions in the C++ Date and Time Library
Some key functions to familiarize yourself with include `time()`, `localtime()`, `gmtime()`, and `mktime()`. The `time()` function returns the current time as a `time_t` object. The `localtime()` and `gmtime()` functions convert a `time_t` object to a `struct tm` representing the local time and Greenwich Mean Time (GMT), respectively. The `mktime()` function converts a `struct tm` object to a `time_t` object, which is useful for calculating the number of seconds since the epoch for a specific date and time.Calculating the Day of the Week
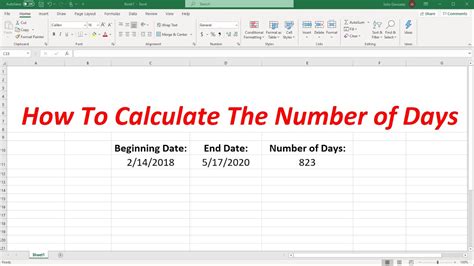
Calculating the day of the week for a given date is a common task in calendar programming. While the localtime()
and gmtime()
functions provide the day of the week as part of the struct tm
, understanding how to manually calculate it can be useful for educational purposes or when working with dates outside the range supported by these functions. Zeller's Congruence is a well-known algorithm for determining the day of the week for any date in the Gregorian calendar.
Implementing Zeller's Congruence
To implement Zeller's Congruence, you need to follow a specific formula that takes into account the year, month, and day of the month. The formula is as follows: `h = (q + [(13*(m+1))/5] + K + [K/4] + [J/4] - 2*J) mod 7`, where `h` is the day of the week (0 = Saturday, 1 = Sunday, ..., 6 = Friday), `q` is the day of the month, `m` is the month (3 = March, 4 = April, ..., 12 = December, 13 = January, 14 = February), `K` is the year of the century (year % 100), and `J` is the century (year / 100).Handling Leap Years
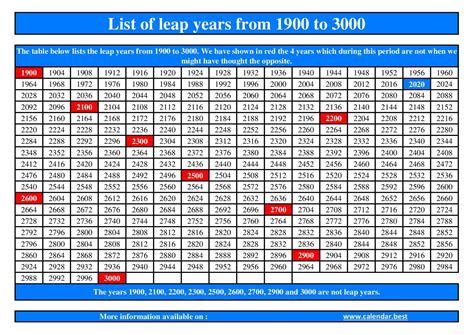
Leap years are a crucial aspect of the Gregorian calendar, which is the most widely used calendar in the world. A leap year occurs every four years to keep the calendar aligned with the Earth's orbit around the Sun. To handle leap years in your calendar applications, you need to determine whether a given year is a leap year or not.
Determining Leap Years
A year is a leap year if it is evenly divisible by 4, except for end-of-century years which must be divisible by 400. This means that the year 2000 was a leap year, although 1900 was not. Implementing this rule in C++ is straightforward and can be done with a simple conditional statement.Creating a Calendar Class
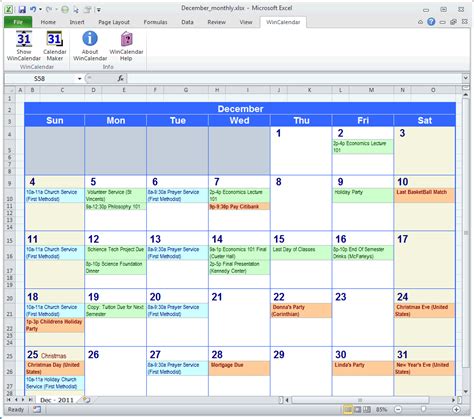
For more complex calendar applications, it's beneficial to create a Calendar
class that encapsulates the functionality of working with dates and times. This class can include methods for calculating the day of the week, determining leap years, and performing other calendar-related operations.
Designing the Calendar Class
When designing the `Calendar` class, consider including methods for setting and getting dates, calculating the number of days between two dates, and checking if a year is a leap year. You can also include overloaded operators to make the class more intuitive to use, such as overloading the `<<` operator to print dates in a human-readable format.Best Practices for Calendar Programming
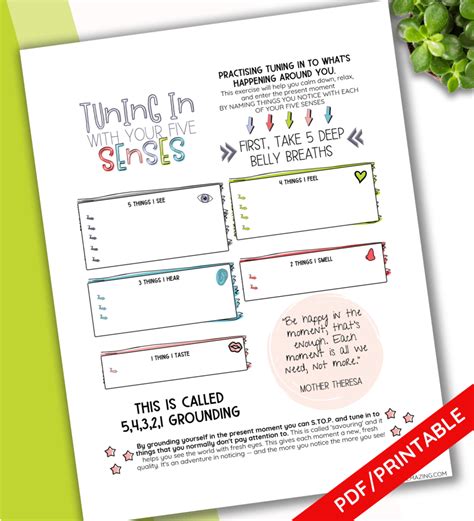
Finally, when programming calendars in C++, it's essential to follow best practices to ensure your code is reliable, maintainable, and efficient. This includes using meaningful variable names, commenting your code, and testing your functions thoroughly.
Testing Calendar Functions
Testing is a critical step in ensuring that your calendar functions work correctly. You should test your functions with a variety of inputs, including edge cases such as February 29th in a leap year and December 31st. Writing unit tests can help automate this process and catch any regressions introduced during future development.Cpp Calendar Image Gallery
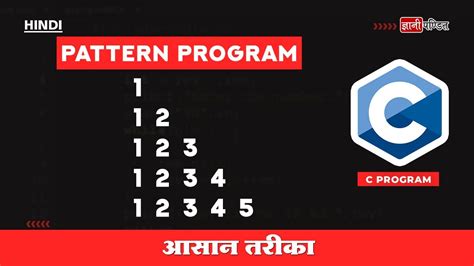
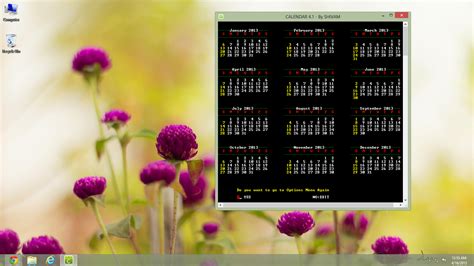
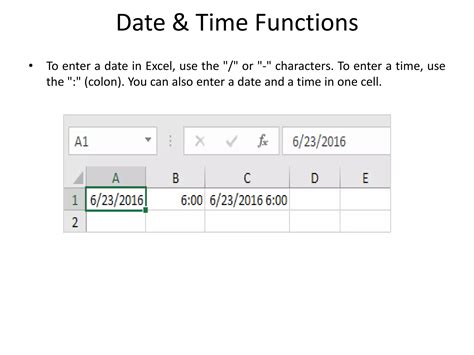
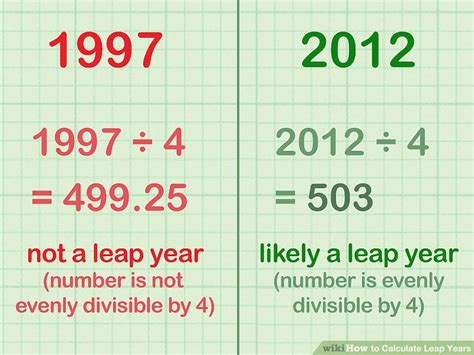
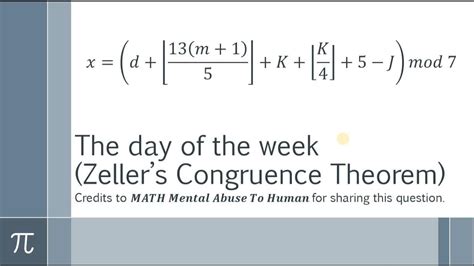
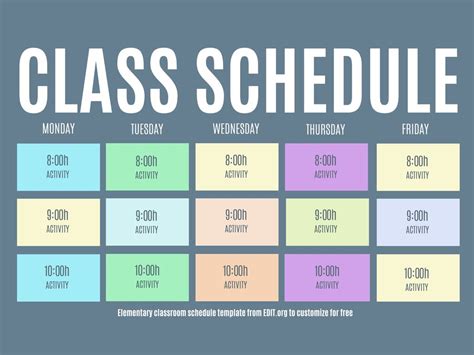
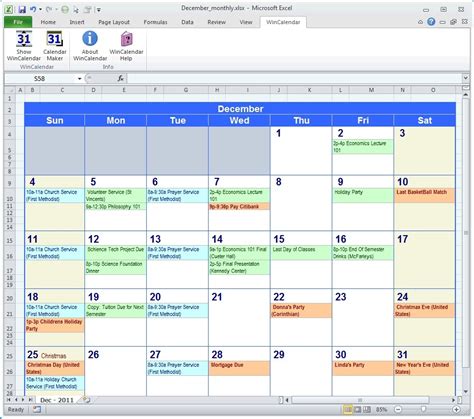
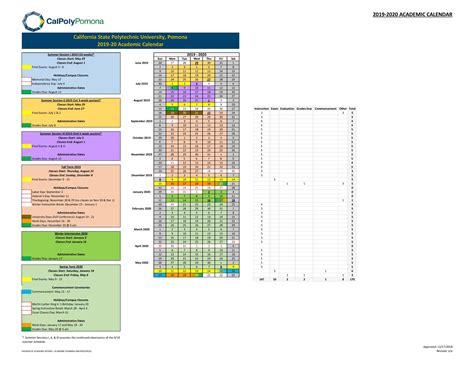
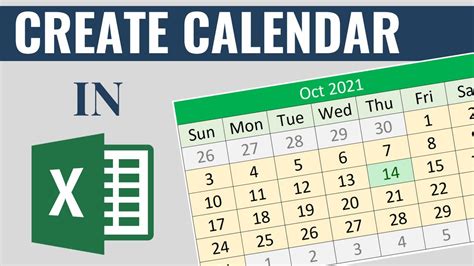
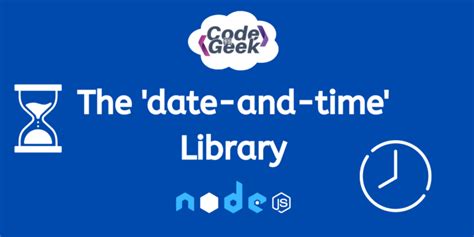
What is the purpose of the `` library in C++?
+
The `
How do you determine if a year is a leap year in C++?
+A year is a leap year if it is evenly divisible by 4, except for end-of-century years which must be divisible by 400.
What is Zeller's Congruence used for in calendar programming?
+Zeller's Congruence is an algorithm used to calculate the day of the week for any date in the Gregorian calendar.
In conclusion, programming calendars in C++ requires a deep understanding of date and time manipulation, as well as the ability to apply algorithms like Zeller's Congruence for calculating the day of the week. By following the tips outlined in this article, including understanding the C++ date and time library, calculating the day of the week, handling leap years, creating a calendar class, and following best practices, you can develop robust and efficient calendar applications. Whether you're building a simple scheduler or a complex event management system, mastering calendar programming in C++ will significantly enhance your skills as a programmer. We invite you to share your experiences and tips for working with calendars in C++, and to explore the many resources available for further learning and development in this fascinating area of programming.