Intro
Learn C++ calendar implementation with this guide, covering date classes, time zones, and scheduling algorithms for efficient programming and development.
The C++ programming language provides a robust foundation for creating complex applications, including those that require date and time management. A calendar implementation in C++ can be a valuable tool for various tasks, such as scheduling, event planning, and data analysis. In this article, we will delve into the world of C++ calendar implementation, exploring its importance, benefits, and key considerations.
C++ is a versatile language that allows developers to create customized calendar systems tailored to specific needs. By leveraging the language's object-oriented programming (OOP) features, developers can design and implement calendar classes that encapsulate date and time logic. This approach enables the creation of reusable and maintainable code, making it easier to integrate calendar functionality into larger applications.
The importance of a well-implemented calendar system cannot be overstated. In many industries, such as finance, healthcare, and transportation, accurate date and time management is crucial for ensuring compliance with regulations, scheduling appointments, and coordinating events. A C++ calendar implementation can help developers create reliable and efficient date and time management systems, reducing the risk of errors and improving overall productivity.
Introduction to C++ Calendar Implementation
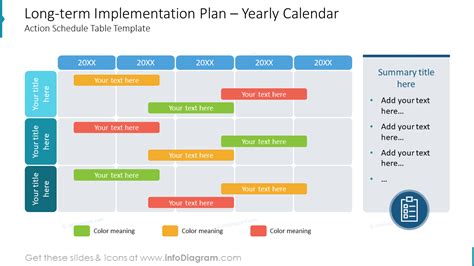
To create a C++ calendar implementation, developers need to consider several key factors, including date and time representation, leap year handling, and timezone support. The C++ Standard Library provides various classes and functions for working with dates and times, such as the std::time_t
and std::tm
structures. However, these classes may not provide the level of customization required for complex calendar systems.
Benefits of C++ Calendar Implementation
The benefits of a C++ calendar implementation are numerous. Some of the most significant advantages include:- Customization: C++ allows developers to create tailored calendar systems that meet specific requirements.
- Reusability: By encapsulating date and time logic in reusable classes, developers can reduce code duplication and improve maintainability.
- Efficiency: A well-implemented C++ calendar system can provide fast and accurate date and time calculations, reducing the risk of errors and improving overall performance.
- Flexibility: C++ calendar implementations can be easily integrated into various applications, from command-line tools to graphical user interfaces.
C++ Calendar Implementation Basics
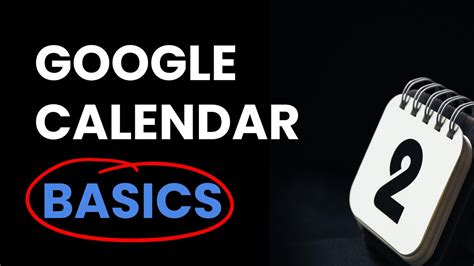
To create a basic C++ calendar implementation, developers need to understand the fundamentals of date and time representation. In C++, dates and times are typically represented using the std::time_t
and std::tm
structures. The std::time_t
structure represents a point in time as a 64-bit integer, while the std::tm
structure provides a more detailed representation of a date and time, including the year, month, day, hour, minute, and second.
C++ Calendar Implementation Steps
The following steps provide a general outline for creating a C++ calendar implementation:- Define a date and time representation: Choose a suitable date and time representation, such as the
std::time_t
andstd::tm
structures. - Implement leap year handling: Develop a mechanism for handling leap years, which occur every 4 years.
- Add timezone support: Incorporate timezone support to ensure accurate date and time calculations across different regions.
- Create calendar classes: Design and implement calendar classes that encapsulate date and time logic, providing methods for date and time calculations, formatting, and parsing.
- Test and refine the implementation: Thoroughly test the C++ calendar implementation to ensure accuracy and reliability, refining the implementation as needed.
Advanced C++ Calendar Implementation Topics
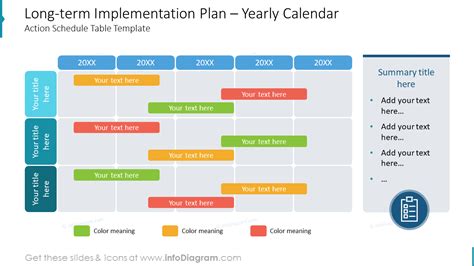
In addition to the basic concepts, there are several advanced topics to consider when creating a C++ calendar implementation. These include:
- Date and time formatting: Providing methods for formatting dates and times in various formats, such as ISO 8601 or RFC 2822.
- Date and time parsing: Implementing methods for parsing dates and times from strings or other input formats.
- Timezone arithmetic: Developing mechanisms for performing timezone-aware arithmetic operations, such as calculating the difference between two dates and times in different timezones.
- Calendar systems: Supporting multiple calendar systems, such as the Gregorian, Julian, or Hebrew calendars.
C++ Calendar Implementation Best Practices
To ensure a robust and maintainable C++ calendar implementation, follow these best practices:- Use object-oriented programming (OOP) principles to encapsulate date and time logic in reusable classes.
- Leverage the C++ Standard Library's date and time classes and functions, such as
std::time_t
andstd::tm
. - Provide thorough documentation and testing to ensure accuracy and reliability.
- Consider using existing C++ calendar libraries or frameworks to simplify the implementation process.
C++ Calendar Implementation Examples
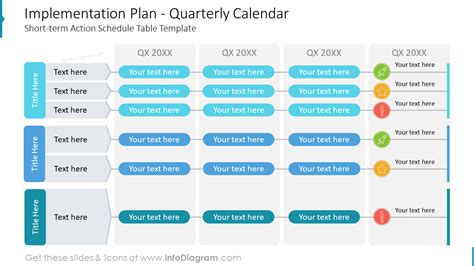
To illustrate the concepts and techniques discussed in this article, consider the following C++ calendar implementation examples:
- A simple command-line calendar application that displays the current date and time.
- A graphical user interface (GUI) calendar application that allows users to schedule appointments and events.
- A web-based calendar application that provides timezone-aware date and time calculations and formatting.
C++ Calendar Implementation Challenges
While creating a C++ calendar implementation can be a rewarding experience, there are several challenges to overcome. These include:- Handling leap years and timezone transitions correctly.
- Providing accurate and efficient date and time calculations.
- Supporting multiple calendar systems and date and time formats.
- Ensuring thread-safety and reentrancy in multi-threaded environments.
C++ Calendar Implementation Image Gallery
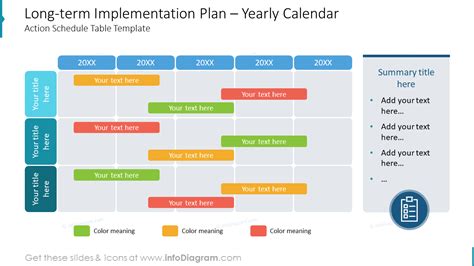
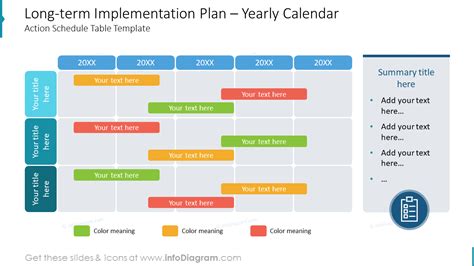
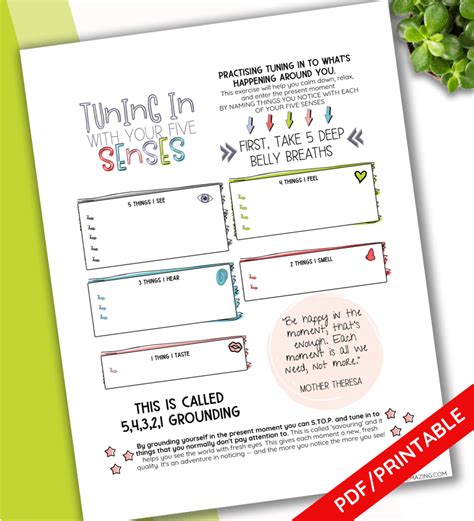
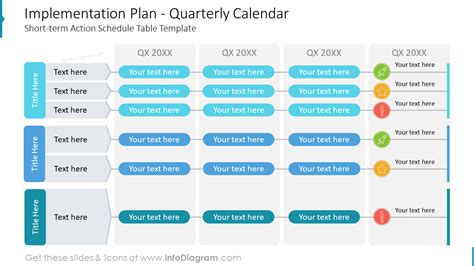
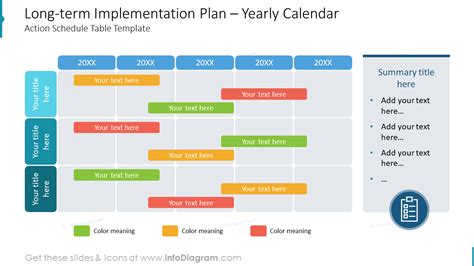
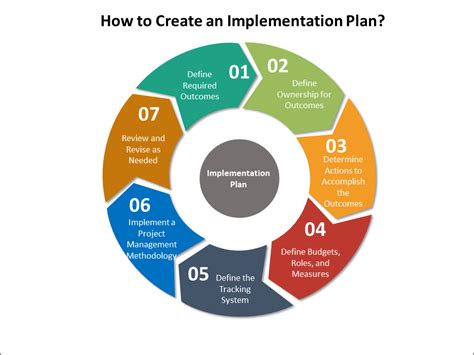
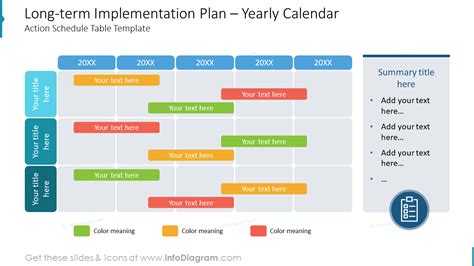
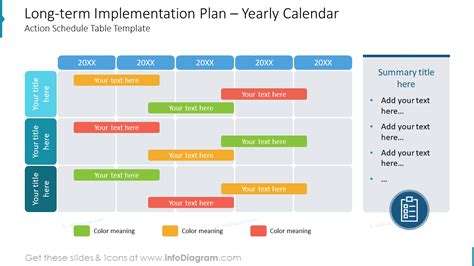
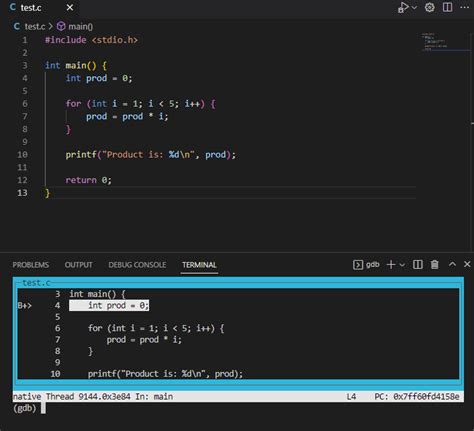
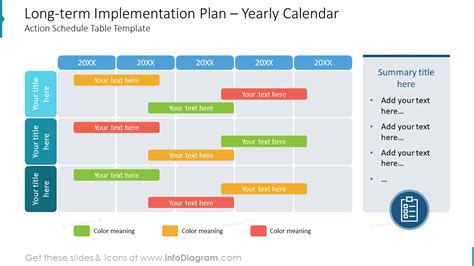
What is the purpose of a C++ calendar implementation?
+The purpose of a C++ calendar implementation is to provide a robust and efficient date and time management system for various applications, including scheduling, event planning, and data analysis.
What are the benefits of a C++ calendar implementation?
+The benefits of a C++ calendar implementation include customization, reusability, efficiency, and flexibility, making it an ideal solution for complex date and time management tasks.
How do I implement a C++ calendar system?
+To implement a C++ calendar system, follow these steps: define a date and time representation, implement leap year handling, add timezone support, create calendar classes, and test and refine the implementation.
What are some advanced C++ calendar implementation topics?
+Advanced C++ calendar implementation topics include date and time formatting, date and time parsing, timezone arithmetic, and calendar systems, such as the Gregorian, Julian, or Hebrew calendars.
What are some best practices for C++ calendar implementation?
+Best practices for C++ calendar implementation include using object-oriented programming (OOP) principles, leveraging the C++ Standard Library's date and time classes and functions, providing thorough documentation and testing, and considering existing C++ calendar libraries or frameworks.
In conclusion, a C++ calendar implementation is a valuable tool for managing dates and times in various applications. By understanding the importance, benefits, and key considerations of C++ calendar implementation, developers can create robust and efficient date and time management systems. Whether you're a seasoned C++ developer or just starting out, this article has provided a comprehensive guide to help you navigate the world of C++ calendar implementation. We invite you to share your thoughts, ask questions, and explore the possibilities of C++ calendar implementation in the comments below.